🛠️ 1-Minute Fixes for .NET Devs
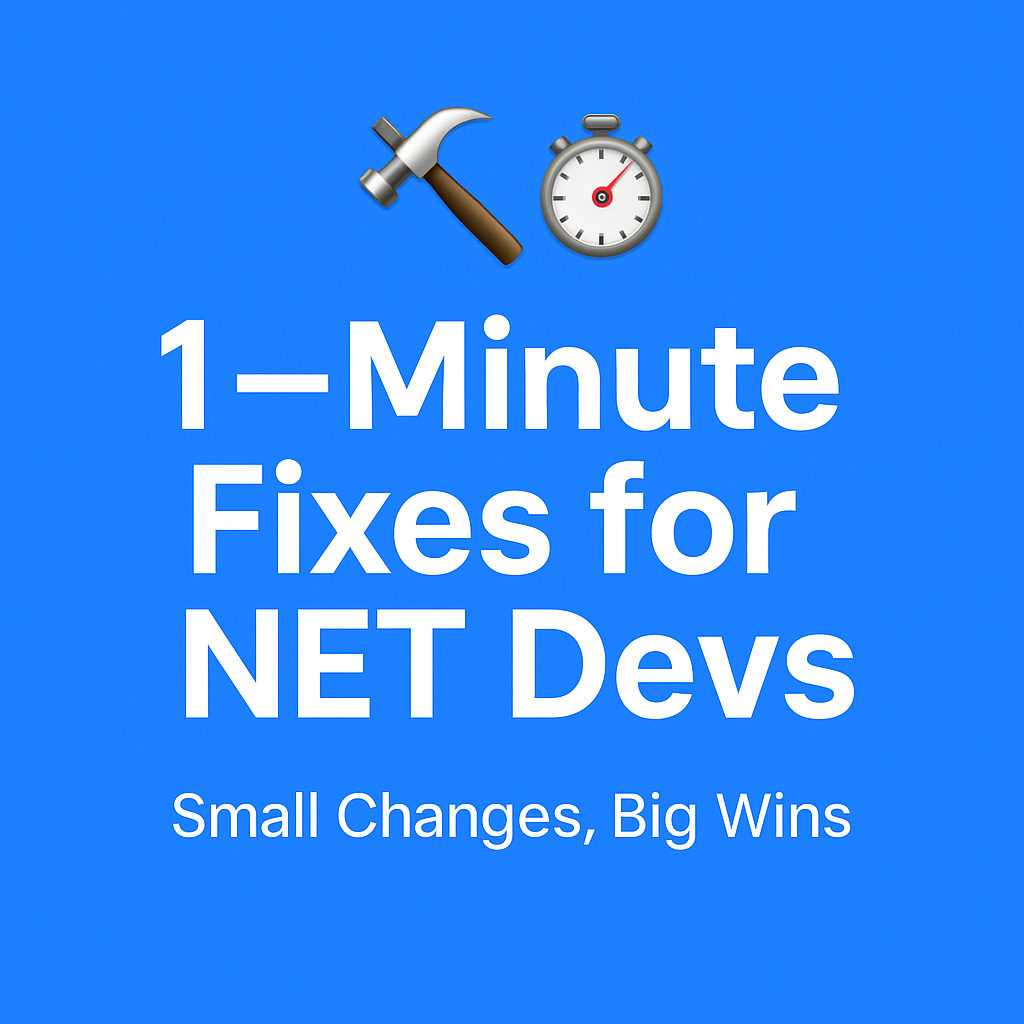
“Sometimes, all it takes is 60 seconds to save hours of debugging.”
✅ 1. Fix Null Reference Exceptions with ?.
Before:
var name = user.Address.City.ToUpper(); // Boom!
✅ After (Safe Call)
var name = user?.Address?.City?.ToUpper();
💡 Why?
Avoid runtime crashes. This is the safest way to deal with potentially null objects without blowing up your app.
✅ 2. Add ConfigureAwait(false)
in Libraries
❌ Before:
await SomeAsyncMethod();
✅ After:
await SomeAsyncMethod().ConfigureAwait(false);
💡 Why?
Prevents deadlocks in background services, especially in ASP.NET. Keeps your async code lean and mean.
✅ 3. Log the Exception Object, Not Just the Message
❌ Before:
_logger.LogError(ex.Message);
✅ After:
_logger.LogError(ex, "An error occurred");
💡 Why?
You get the full stack trace, inner exceptions, and helpful context. Never log half the truth.
✅ 4. Don’t Use DateTime.Now
for Business Logic
Instead, use DateTime.UtcNow
or inject an IDateTimeProvider
public interface IDateTimeProvider {
DateTime UtcNow { get; }
}
💡 Why?
Ensures consistency in testing, logging, and time zone independence. Your code behaves the same everywhere.
🧠 Bonus Tips (Write these on a sticky note!)
- Stop swallowing exceptions silently. Future you will curse you.
- Rename
Utils.cs
to something that makes sense. - Don’t abuse
var
. Be expressive where it matters. - Log at the right level. Debug ≠ Info ≠ Error.
✍️ Final Thoughts
These aren’t massive refactors or sweeping redesigns—
They’re simple, proven fixes that add up to better software and fewer 2 AM incidents.
Clean code starts with small wins. Why not get one in the next minute?
📣 Call to Action
🔥 Save this. Share with your team.
🎯 Turn it into a personal checklist.
💬 Got your own 1-minute tip? Drop it in the comments!