β Common EF Core LINQ Mistakes to Avoid for Better Performance π«
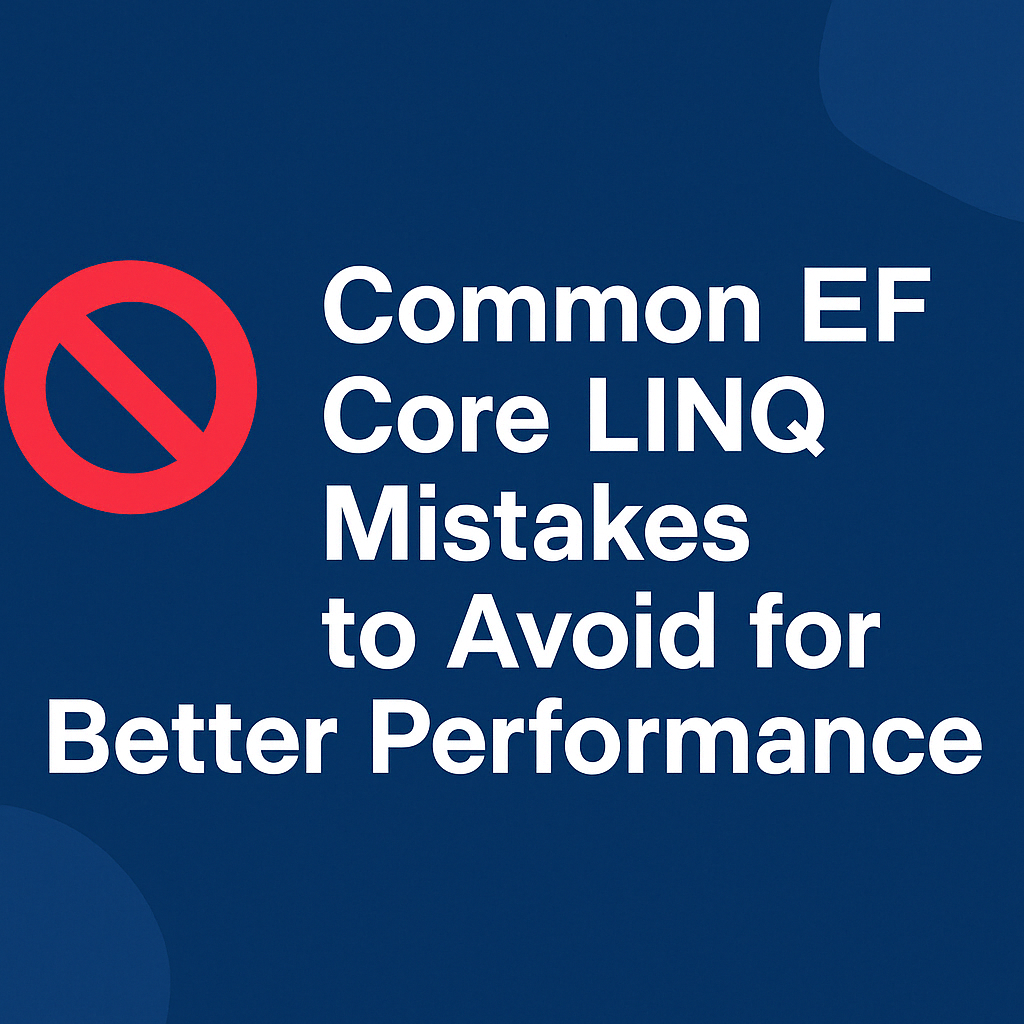
Writing LINQ with Entity Framework Core is powerful β but small mistakes can lead to serious performance hits, data issues, and runtime exceptions. Here's a list of real-world mistakes developers commonly make (and how to avoid them) when working with EF Core LINQ.
1οΈβ£ Calling .ToList()
Too Early π
Mistake: Fetching data into memory before filtering.
Why it's bad: Increases memory usage and queries unnecessary data from the database.
// β Inefficient
var users = db.Users.ToList().Where(u => u.IsActive);
// β
Efficient
var users = db.Users.Where(u => u.IsActive).ToList();
2οΈβ£ Forgetting .AsNoTracking()
on Read-Only Queries π§Ύ
Mistake: Using default tracking for queries that donβt need updates.
Why it's bad: Adds unnecessary memory overhead, which impacts performance when you're only reading data.
// β
Recommended for read-only queries
var users = db.Users.AsNoTracking().ToList();
π§ Tip: Use .AsNoTracking()
for queries where you don't plan to update the entities. It keeps your DbContext lightweight and improves read performance.
3οΈβ£ Using Include()
Without Needing the Related Data π¦
Mistake: Eager loading navigation properties even when not used.
Why it's bad: Bloats queries, increases data transfer size, and slows down performance.
// β Donβt use Include if you don't need related data
var orders = db.Orders.Include(o => o.Customer).ToList();
π§ Tip: Only use .Include()
when you're going to access the related data. Otherwise, stick to lazy loading or just avoid loading unused relationships.
4οΈβ£ Querying Navigation Collections in Loops (N+1 Problem) π
Mistake: Running queries inside loops for related data.
Why it's bad: Sends multiple queries to the database β one per item in the loop β drastically slowing down your app (the classic N+1 problem).
// β Triggers a query per user
foreach (var user in db.Users.ToList())
{
var orders = db.Orders.Where(o => o.UserId == user.Id).ToList();
}
// β
Optimized with eager loading
var usersWithOrders = db.Users.Include(u => u.Orders).ToList();
5οΈβ£ Not Using Projections with .Select()
π―
Mistake: Fetching full entities when only specific fields are needed.
Why it's bad: Loads unnecessary data into memory, increasing query time and memory usage β especially with large datasets.
// β Loads all fields
var users = db.Users.Where(u => u.IsActive).ToList();
// β
Loads only what's needed
var userSummaries = db.Users
.Where(u => u.IsActive)
.Select(u => new { u.Name, u.Email })
.ToList();
π§ Tip: Use .Select()
to project only the fields you need. It makes queries faster and keeps your application lean.
6οΈβ£ Using Count()
Instead of Any()
for Existence Checks π
Mistake: Checking for the existence of records using .Count() > 0
.
Why it's bad: .Count()
scans all matching records, even after finding the first. .Any()
short-circuits and stops once it finds a match β making it much faster.
// β Slower
if (db.Users.Where(u => u.IsActive).Count() > 0)
// β
Faster
if (db.Users.Any(u => u.IsActive))
π§ Tip: Use .Any()
when you're checking if at least one record exists. It's optimized for performance and is the correct semantic approach.
7οΈβ£ Mixing IQueryable
with IEnumerable
Too Soon β οΈ
Mistake: Materializing data early and filtering in memory.
Why it's bad: You lose the benefits of SQL-side filtering, resulting in slower performance on large datasets.
// β In-memory filtering
var data = db.Users.ToList().Where(u => u.IsActive);
// β
DB-side filtering
var data = db.Users.Where(u => u.IsActive).ToList();
8οΈβ£ Writing Logic EF Core Canβt Translate to SQL β
Mistake: Using methods in LINQ that EF Core can't convert to SQL.
Why it's bad: Causes runtime exceptions or forces client-side evaluation, which can crash your app or severely degrade performance.
// β Wonβt work if MyCustomMethod is not translatable
var users = db.Users.Where(u => MyCustomMethod(u.Name)).ToList();
9οΈβ£ Not Disposing DbContext
Properly π₯
Mistake: Keeping DbContext
alive for too long or forgetting to dispose of it.
Why it's bad: Can lead to memory leaks, connection pool exhaustion, and unstable application behavior.
// β
Always dispose properly
using(var context = new MyDbContext())
{
var users = context.Users.ToList();
}
π Not Reviewing Generated SQL β
Mistake: Assuming LINQ always generates optimized SQL.
Why it's bad: You might be executing inefficient joins, nested subqueries, or pulling unnecessary columns without realizing it.
// β
Use ToQueryString() to inspect generated SQL
var query = db.Users.Where(u => u.IsActive);
Console.WriteLine(query.ToQueryString());
β Final Thoughts
Entity Framework Core makes querying intuitive β but one wrong move can kill your performance or crash production. Avoiding these common EF Core LINQ mistakes will help you write faster, cleaner, and more maintainable code.
π‘ Pro Tip: Always profile your SQL, avoid unnecessary data loads, and prefer server-side execution whenever possible!