๐ Folder-by-Folder Breakdown of a Scalable .NET Project (Clean Architecture Style)
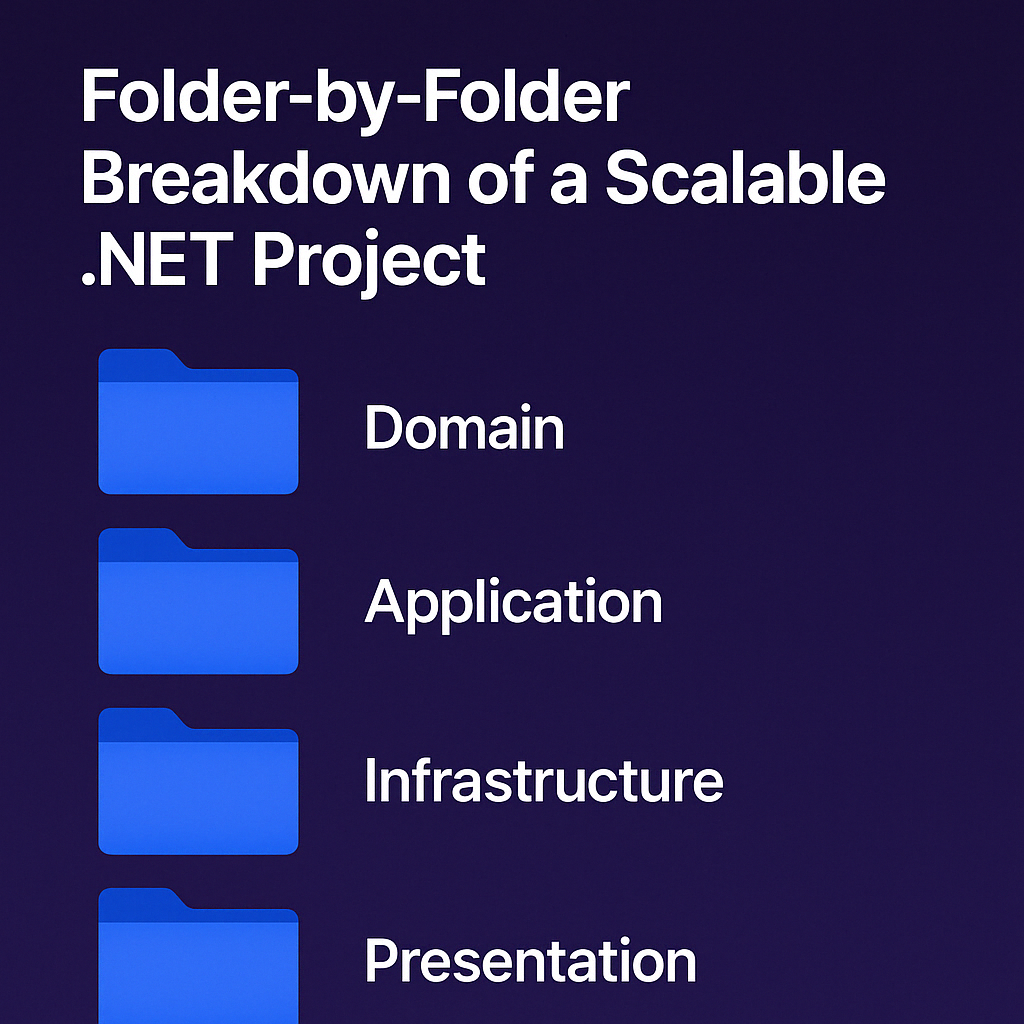
Day 2 โ Wednesday: The Backbone of a Maintainable Application
Welcome back, devs!
On Day 1, we explored what Clean Architecture is and why it actually matters in the long run.
Today, we go hands-on.
In this post, Iโll walk you through how to structure your .NET application using Clean Architecture principlesโfolder by folder, and explain what goes where and why. We'll also visualize how these layers talk to each other.
Letโs dive in. ๐
๐งฑ High-Level Overview of Clean Architecture Layers
Here's the folder layout at a glance:
Each layer has a responsibility, and no layer leaks into the one below it. Dependency always flows inward.
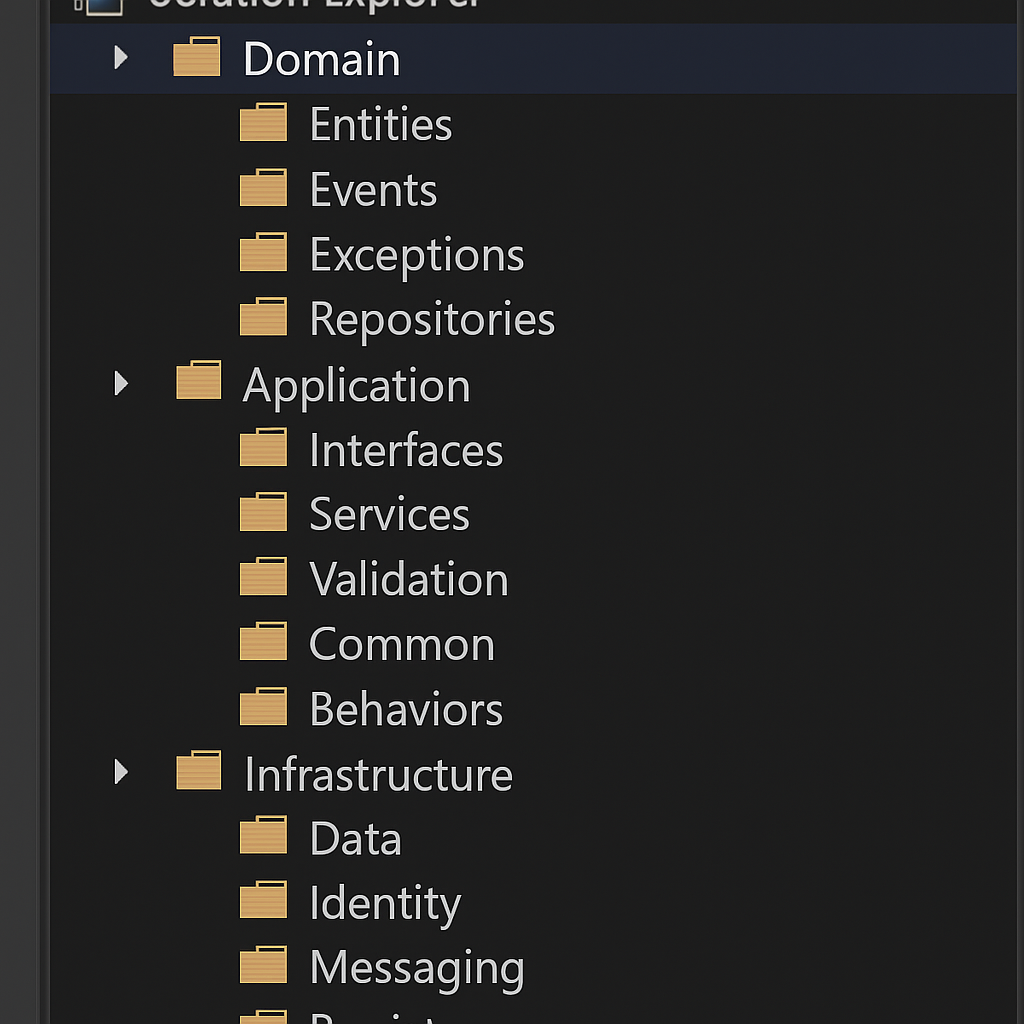
๐ Domain โ โThe Heart of the Appโ
"This is your pure logic โ untouched by frameworks or databases."
Purpose: Defines the core business entities, rules, and contracts.
What goes here?
Entities
: POCO classes likeProduct
,Order
, etc.ValueObjects
: Immutable types likeEmail
,Money
, etc.Enums
: Domain-specific enumerations.Interfaces
: Abstract definitions that Infrastructure or Application will implement.
๐ Application โ โThe Use Case Layerโ
"This is the brain. It knows what to do, but not how to do it."
Purpose: Orchestrates use cases and application logic.
What goes here?
Interfaces
: Contracts for services and repositories.Services
: Use case handlers (ProductService
,OrderProcessor
).DTOs
: Data transfer objects for input/output.
๐ Infrastructure โ โThe Tools & Wiringโ
"This is where implementation details live โ EF Core, file systems, APIs."
Purpose: Implements interfaces defined in Application and Domain.
What goes here?
Data
: EF CoreDbContext
, repositories.Services
: Implementations of interfaces (e.g.,EmailSender
,FileStorage
).- Third-party integrations: payment gateways, cloud APIs, etc.
๐ Presentation / WEB UIโ โThe UI or API Layerโ
"The outer shell that talks to the world."
Purpose: This layer exposes your app to the outside world โ via APIs or UI.
What goes here?
Controllers
: REST API endpoints for consumersFilters/Middleware
: Logging, authentication, global error handling- Model binding and validation
- ๐ Bonus: How the Layers Talk to Each Other
Hereโs a quick visualization of dependency direction:
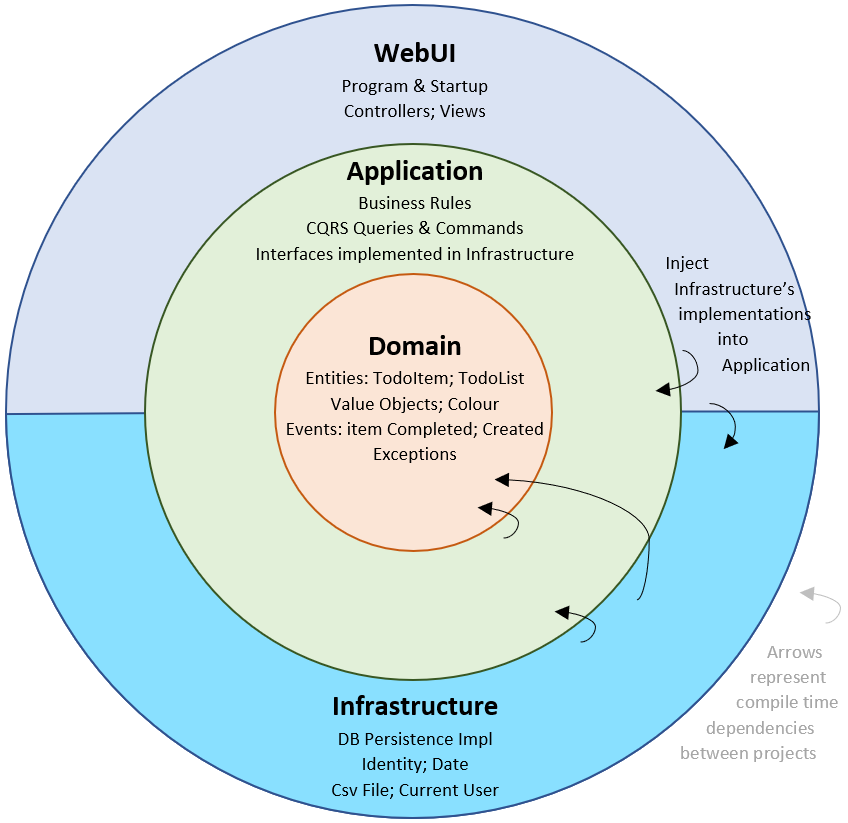
- โ Domain: No dependencies.
- โ Application: Only depends on Domain.
- โ Infrastructure: Implements Application and Domain interfaces.
- โ Presentation: Knows only about Application.
๐งญ This makes it easy to swap your UI, database, or services without affecting your business rules.
๐ง Why This Structure Helps You Scale
- ๐ Swap implementations easily (e.g., change database provider).
- ๐งช Test business logic without touching controllers or DB.
- ๐งน Keep code clean, modular, and understandable.
- ๐ Makes onboarding new devs way easier.
๐ง Final Thoughts
As youโve seen today, Clean Architecture isnโt just a buzzword โ itโs a strategy that protects your app from chaos as it grows.
The moment you separate concerns and isolate dependencies using these four core layers โ Domain, Application, Infrastructure, and Presentation โ your codebase becomes more testable, flexible, and easier to understand.
Whether you're building a side project or an enterprise application, this structure gives you the clarity and control you need to scale with confidence.
๐ End Note
In our next post (๐ Day 3 ), weโll explore how to bring SOLID principles to life inside your Application and Domain layers โ with real-world C# examples and practical tips for staying clean and composable.
Until then:
- ๐ Share this post if you found it helpful
- ๐ฌ Drop your thoughts or questions in the comments
- ๐ง And most importantly โ keep learning, building, and writing clean code!
Until then, happy coding and keep it clean! โจ