π Mastering MassTransit in .NET 9: The Modern Dev's Guide to Scalable Messaging
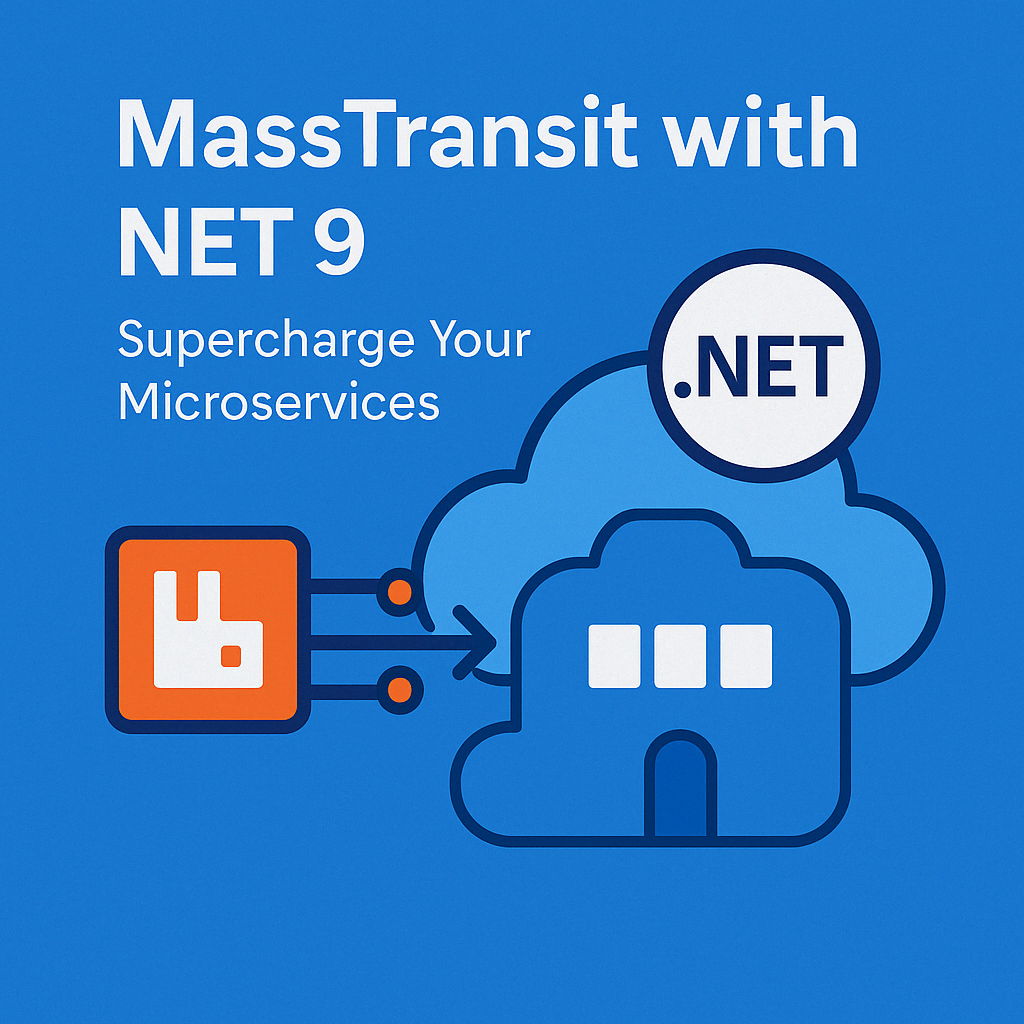
Tired of boilerplate event bus blogs? This one's different. Let's make messaging β¨magicalβ¨ with MassTransit.
π What Is MassTransit & Why Should You Care?
MassTransit is a powerful, open-source distributed application framework for .NET. It abstracts messaging systems like RabbitMQ, Azure Service Bus, and Kafka β so you can build message-driven microservices without reinventing the queue.
Whether you're building enterprise microservices or just want clean separation of concerns β MassTransit is your friend.
βοΈ How to Use MassTransit in .NET 9 (w/ RabbitMQ)
π¦ Step 1: Install NuGet Packages
Start by installing the required MassTransit packages for RabbitMQ integration:
dotnet add package MassTransit
dotnet add package MassTransit.RabbitMQ
π₯ Quick Tip: Run these commands in your project directory to add the libraries to your .csproj
file.
Visit the link to know more about available Packages : https://masstransit.io/support/packages
π§ Configure Services
Now configure MassTransit and register your consumer using RabbitMQ in the Program.cs
file (with .NET 9 minimal hosting):
// Program.cs (.NET 9 minimal hosting)
builder.Services.AddMassTransit(x =>
{
x.AddConsumer<OrderCreatedConsumer>();
x.UsingRabbitMq((context, cfg) =>
{
cfg.Host("rabbitmq://localhost");
cfg.ConfigureEndpoints(context);
});
});
β‘ Pro Tip: ConfigureEndpoints(context)
auto-binds consumers to their respective queues based on convention β no manual queue naming needed!
π© Define the Message Contract
When working with MassTransit in a .NET 9 application, defining message contracts as interfaces helps decouple producers and consumers. Here's how you can define a simple contract for an order creation event:
public interface IOrderCreated
{
Guid OrderId { get; }
DateTime CreatedAt { get; }
}
β Tip: Keep your message contracts in a shared project or library that's referenced by both the producer and consumer services.
π οΈ Create a Consumer
Consumers in MassTransit handle incoming messages and execute business logic. Here's how to create a consumer that listens for the IOrderCreated
event:
public class OrderCreatedConsumer : IConsumer<IOrderCreated>
{
public Task Consume(ConsumeContext<IOrderCreated> context)
{
Console.WriteLine($"Order Received: {context.Message.OrderId}");
return Task.CompletedTask;
}
}
π Note: You can inject services via constructor to process orders, update databases, or trigger workflows.
π Publish the Message
Publishing a message in MassTransit is straightforward and follows a strongly-typed contract-first approach. Here's how you can publish the IOrderCreated
message:
await bus.Publish<IOrderCreated>(new
{
OrderId = Guid.NewGuid(),
CreatedAt = DateTime.UtcNow
});
π§ Dev Tip: Use strongly typed contracts for better debugging, IntelliSense support, and long-term scalability.
βοΈ MassTransit vs Other Messaging Libraries
Feature | MassTransit | MediatR | Raw RabbitMQ Client |
---|---|---|---|
Abstraction Level | High | Internal CQRS only | Low |
Broker Support | RabbitMQ, Azure SB, Kafka | N/A | RabbitMQ only |
Outbox Support | Yes (EFCore, Mongo) | No | No |
Saga State Machines | Built-in | No | Manual |
Middleware/Filters | Supported | Partial | Manual |
Production Readiness | β Yes | β οΈ Limited | β οΈ Requires plumbing |
β‘ Hot Take: MassTransit gives you 80% power with 20% complexity β perfect for real microservices.
β¨ Why Devs Love MassTransit
- Scalable Messaging with minimal boilerplate
- Built-in Retry, Circuit Breakers, Rate Limiting
- Saga and State Machine Support
- Easy to plug into DI in .NET 9
- Open Source and Actively Maintained
β οΈ Things to Watch Out For
- Steep Learning Curve for advanced concepts like sagas
- Higher Memory Footprint compared to leaner libraries
- Requires a Message Broker (e.g., RabbitMQ setup)
β οΈ Pro Tip: Use MassTransit only when your app truly benefits from message-driven architecture. Donβt over-engineer!
π οΈ Real-World Use Cases
- E-commerce: Handle payment, order, and shipment flows
- IoT: Process sensor data asynchronously
- Finance Apps: Decouple fraud detection pipelines
- Workflow Orchestration: Use Sagas to model stateful processes
β Final Thoughts
MassTransit is not just another library β itβs a full messaging framework that brings out the best in your distributed .NET apps.
If you're serious about building resilient, event-driven systems, itβs one of the most powerful tools in your kit.
Next Step? Try building a checkout workflow using MassTransit sagas. Game changer!
π§© Bonus: EF Core Outbox Pattern with MassTransit
To ensure message consistency in distributed systems, use the Outbox Pattern with MassTransit and Entity Framework. This guarantees that your database changes and message publishing occur atomically.
builder.Services.AddMassTransit(x =>
{
x.AddEntityFrameworkOutbox<ApplicationDbContext>(o =>
{
o.UsePostgres();
o.UseBusOutbox(); // crucial for atomicity
});
});
βοΈ Pro Tip: UseBusOutbox()
ensures that your messages are only published
π Useful Resources
Thanks for sticking around, devs! π―
MassTransit might seem complex at first, but once you see it in action, it opens up a world of scalable, event-driven architecture. As .NET 9 pushes the boundaries of modern development, tools like MassTransit help you stay ahead of the curve.
π₯ Want to go deeper? In upcoming posts, weβll explore Saga State Machines, Retry Policies, and Outbox integrations in real-world enterprise applications.
Stay tuned β and happy coding! π»β¨
π¬ Got questions or success stories with MassTransit? Drop them in the comments β letβs build better systems together!