π Top 5 EF Core LINQ Features You Should Be Using in 2025
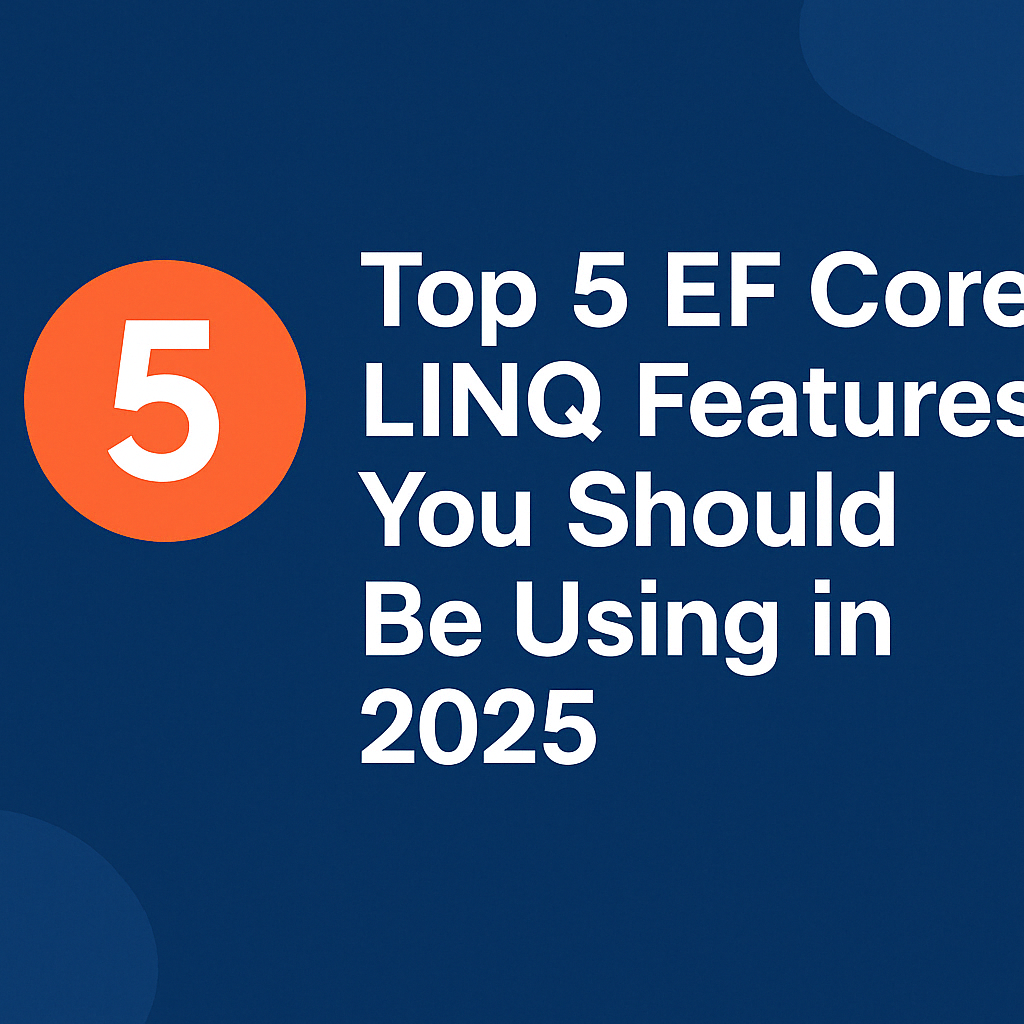
Supercharge your .NET apps with these powerful and modern EF Core LINQ features. From SQL-like pattern matching to bulk updates β these features are essential for clean and performant code. πͺ
1οΈβ£ Use EF.Functions.Like
for Smart SQL Pattern Matching π‘
Use SQL LIKE
directly in LINQ for flexible wildcard searches without raw SQL.
var users = db.Users
.Where(u => EF.Functions.Like(u.Email, "%@gmail.com"))
.ToList();
2οΈβ£ Access Dynamic or Shadow Properties with EF.Property<T>()
π
Retrieve unmapped or dynamically named column values safely within LINQ.
var result = db.Entries
.Where(e => EF.Property<string>(e, "Status") == "Approved")
.ToList();
3οΈβ£ Debug Smarter with ToQueryString()
π
Get the actual SQL query generated by EF Core before executing β great for debugging and performance reviews.
var query = db.Users.Where(u => u.IsActive);
Console.WriteLine(query.ToQueryString());
4οΈβ£ Update/Delete in Bulk with ExecuteUpdateAsync
& ExecuteDeleteAsync
β‘
Perform batch operations directly in SQL without loading entities into memory. (Available in EF Core 7+)
await db.Users
.Where(u => u.IsInactive)
.ExecuteDeleteAsync();
5οΈβ£ Boost Query Speed with GroupBy Translation Improvements π§
Group and aggregate data directly in SQL, eliminating slow client-side grouping.
var grouped = await db.Orders
.GroupBy(o => o.CustomerId)
.Select(g => new { g.Key, Count = g.Count() })
.ToListAsync();
π§ Bonus Tip: Combine Multiple Filters Efficiently with Predicate Logic
Avoid chaining filters that generate nested queries. Combine with cleaner expression logic.
var isRecent = true;
var hasPaid = true;
var orders = db.Orders
.Where(o =>
(!isRecent || o.Date > DateTime.UtcNow.AddDays(-30)) &&
(!hasPaid || o.Status == "Paid"))
.ToList();
β Final Thoughts
These modern LINQ features not only make your EF Core queries elegant, but they also significantly improve performance. Adopt them into your daily development workflow and build cleaner, faster, and more maintainable codebases.